State management is a fundamental aspect of building applications with React. As your application grows, managing state can become complex, so it’s essential to understand the best practices and techniques to handle it efficiently. This article explores the best ways to manage state in React, from local component state to global state management solutions.
Understanding React State
Local State
Local state is confined to a single component and is managed using the useState
hook. It’s suitable for simple use cases where the state doesn’t need to be shared across multiple components. This approach works well for component-specific data and straightforward UI interactions, such as toggling a modal or handling form inputs.
When to Use Local State
- Component-specific data: When the state is only relevant to a single component.
- Simple interactions: For straightforward UI interactions like toggling a modal or handling form inputs.
Context API for Global State
The Context API is a built-in solution for sharing state across multiple components without the need for prop drilling.
Creating and Using Context
To use the Context API, you create a context and provide it to the components that need access to the shared state. This involves creating a context, a provider component that wraps the parts of your app that need access to the context, and using the useContext
hook to access the context value within your components.
When to Use Context API
- Global data: When the state needs to be accessed by many components at different levels of the component tree.
- Theming and localization: For managing themes, language settings, and user authentication.
Redux for Advanced State Management
Redux is a powerful state management library that provides a predictable way to manage the state of your application. It’s suitable for complex applications where the state management logic becomes intricate.
Setting Up Redux
Setting up Redux involves creating actions and reducers to define how state changes in response to actions, configuring the store to hold your application’s state, and connecting your components to the store using the useSelector
and useDispatch
hooks from the react-redux
library.
When to Use Redux
- Large-scale applications: When the application state is complex and involves many interactions.
- Predictability and debugging: Redux’s strict structure helps in maintaining predictability and makes debugging easier.
Other State Management Solutions
Zustand
Zustand is a small, fast, and scalable state management solution. It’s easy to use and doesn’t require the boilerplate code like Redux. Zustand is ideal for simple to moderately complex state management needs.
Recoil
Recoil is a state management library developed by Facebook. It’s designed to work seamlessly with React’s concurrent mode and is ideal for complex applications that require fine-grained control over state.
When to Use Other Solutions
- Zustand: When you need a simple, fast, and scalable solution without the complexity of Redux.
- Recoil: For complex applications that benefit from its integration with React’s concurrent mode and fine-grained state management.
Enozom is a software development company known for its expertise in creating custom web and mobile applications. In the realm of React development, Enozom excels in managing state efficiently, leveraging various state management solutions to build scalable and maintainable applications. By utilizing techniques such as the Context API for sharing state across components and Redux for handling complex state logic, Enozom ensures that their applications remain robust and performant. Their adept use of modern libraries like Zustand and Recoil further exemplifies their commitment to adopting the best practices in state management, enabling them to deliver high-quality software solutions tailored to their clients’ needs.
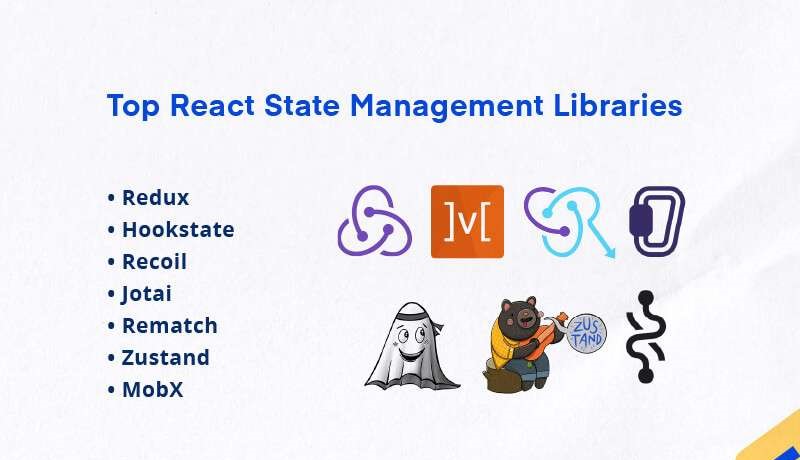
Best Practices for State Management in React
Lift State Up
When multiple components need to share the same state, lift the state up to their nearest common ancestor. This ensures that the state is managed in a single place and can be easily passed down to child components.
Avoid Overusing Context
Use the Context API judiciously. Overusing it can lead to performance issues as every context change triggers a re-render of all consuming components.
Normalize State Shape
In Redux, normalize the state shape to avoid deeply nested structures, making it easier to update and manage.
Use Custom Hooks
Encapsulate complex state logic in custom hooks to make your components cleaner and more reusable. This approach helps in separating state management logic from component logic, enhancing code readability and maintainability.
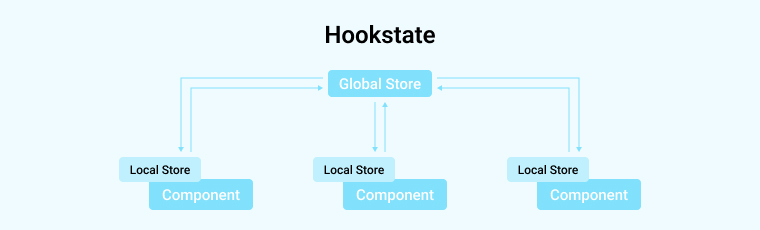
Keep State Minimal
Store only what you need in the state. Derived data should be computed on the fly instead of being stored. This reduces the complexity of your state and makes your application more efficient.
Code Splitting and Lazy Loading
For large applications, split your state management logic into separate modules and use lazy loading to improve performance. This ensures that only the necessary parts of the state management logic are loaded when required, enhancing the overall performance of your application.
Conclusion
Effective state management is crucial for building scalable and maintainable React applications. Depending on your application’s complexity and requirements, you can choose between local state, the Context API, Redux, Zustand, or Recoil. Understanding and applying the right state management techniques and best practices will help you build efficient and robust React applications.