Unit testing is a fundamental practice in modern software development that directly impacts the quality and reliability of software. It provides the confidence to make changes quickly, safely, and accurately. In this article, we’ll take a deep dive into unit testing, exploring practical methods, common strategies, advanced techniques, pitfalls to avoid, and best practices that ensure effective and maintainable tests.
Understanding Unit Testing
Unit testing involves testing individual units or components of software independently to verify they behave as intended. A unit typically refers to the smallest testable part of the software, such as methods or functions. It focuses on isolating a single component and validating its logic in a controlled environment.
Goals of Unit Testing:
- Identify issues early in development.
- Ensure code quality and correctness.
- Simplify debugging and maintenance.
- Facilitate safe refactoring.
Why Unit Testing Is Essential
1. Early Bug Detection
Unit testing allows catching errors early in the development cycle, significantly reducing the time and cost involved in fixing them later.
2. Improved Code Design
Writing testable code encourages modularity and loose coupling, leading to clean and maintainable software architecture.
3. Ease of Refactoring
With comprehensive tests in place, developers can refactor code confidently without fear of introducing regressions or breaking existing functionalities.
4. Documentation and Clarity
Tests can serve as living documentation that clearly demonstrates the expected behavior of your code.
Components of a Good Unit Testing
A robust unit test typically consists of three distinct parts:
- Arrange: Prepare necessary preconditions and inputs.
- Act: Invoke the method under test.
- Assert: Verify the results against expected outcomes.
Example in JavaScript (Jest Framework):
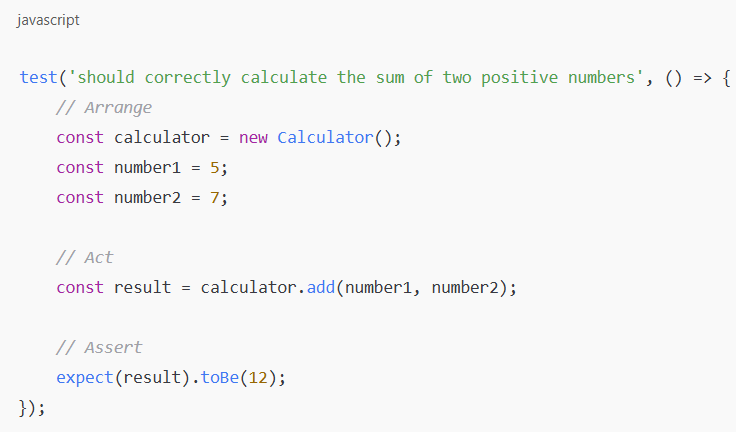
Best Practices for Effective Unit Testing
1. Maintain Isolation
Unit tests should be isolated from external dependencies (databases, network calls, file systems). Using mocks, stubs, or dependency injection enables you to test code independently.
Mocking example using Jest:
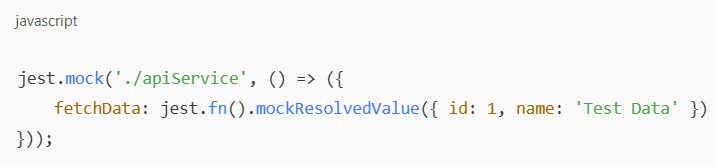
2. Single Responsibility Principle
Each test should verify a single behavior or outcome. Avoid tests that try to validate multiple functionalities simultaneously.
3. Test the Behavior, Not the Implementation
Focus on what the unit should achieve rather than internal details. This practice ensures your tests remain robust even if implementation details change.
4. Clear, Descriptive Test Names
Test names should clearly reflect the scenario under test and its expected outcome, making tests readable and self-explanatory.
Good naming convention example:shouldThrowErrorWhenUserIsNotFound()
Effective Unit Testing Strategies
1. Test-Driven Development (TDD)
TDD is a practice where developers write unit tests before implementing functionality. It follows three steps iteratively:
- Red: Write a failing test first.
- Green: Write minimum necessary code to pass the test.
- Refactor: Refine and improve the code while tests ensure the behavior stays correct.
TDD enforces clarity of requirements and improves overall software design.
2. Boundary and Edge Case Testing
Identify and test boundary values and edge cases, including:
- Empty inputs (
null
, empty strings) - Boundary numbers (
0
,-1
, maximum integer) - Special characters or unusual input data
3. Regression Testing
Regularly running your suite of unit tests after every significant change or feature addition ensures that previously working code still performs correctly.
Advanced Unit Testing Techniques
1. Parameterized Tests
These allow you to run the same test with different input sets.
Example with Jest:
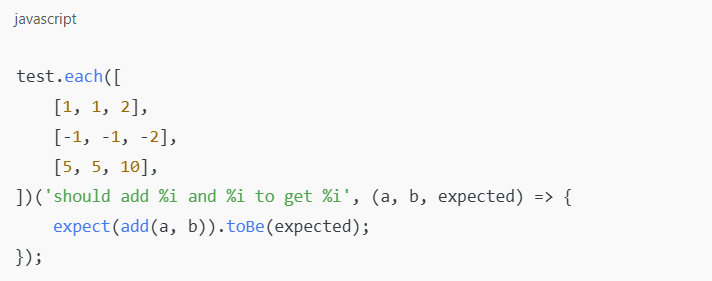
2. Property-Based Testing
Tests code with randomly generated inputs to validate behavior across a wide range of scenarios.
- Tools: QuickCheck (Haskell), Hypothesis (Python), fast-check (JavaScript)
3. Mutation Testing
Mutation testing evaluates your test quality by deliberately introducing faults (mutations) in the code to verify whether your tests can detect them.
- Tools: Stryker (JavaScript/.NET), PIT (Java), Mutmut (Python)
Integrating Unit Testing in CI/CD
Continuous Integration (CI) platforms such as Jenkins, GitLab CI, GitHub Actions, Azure DevOps ensure that unit tests automatically run on each code push, keeping the development pipeline reliable and transparent.
Typical CI steps:
- Check out code
- Run automated tests
- Generate coverage reports
- Notify developers immediately of failures
Code Coverage and Its Misconceptions
Code coverage measures the percentage of your codebase executed by tests. High coverage generally indicates comprehensive tests, but remember:
- High coverage ≠ good quality: Tests must also be meaningful and assert real behaviors.
- Target areas with complex logic and high business impact rather than pursuing coverage blindly.
Recommended Coverage Thresholds:
- New projects: 70-80%
- Critical or core modules: 90-100%
Common Pitfalls and How to Avoid Them
1. Writing Brittle Tests
Avoid tightly coupling your tests to implementation details. Instead, focus on testing outcomes.
2. Ignoring Test Failures
Fix failing tests immediately to maintain confidence in your test suite.
3. Writing Tests After Implementation
Delayed tests tend to become confirmation tests rather than genuinely validating correctness.
Popular Unit Testing Frameworks by Language
- Java: JUnit, TestNG
- JavaScript/TypeScript: Jest, Mocha, Jasmine, Vitest
- Python: unittest, pytest
- .NET: NUnit, xUnit.net, MSTest
- Ruby: RSpec, Minitest
- PHP: PHPUnit
- Flutter/Dart: flutter_test, mockito
Creating a Culture of Unit Testing in Your Team
- Lead by Example: Senior developers and team leads should actively write and maintain tests.
- Educate Your Team: Regularly conduct workshops and encourage knowledge-sharing on best practices and testing techniques.
- Make Testing Part of the Workflow: Integrate unit tests into code reviews, sprints, and retrospectives.
- Encourage Ownership: Each developer should own the tests related to their code.
Measuring Unit Testing Effectiveness
- Test Execution Speed: Tests should run quickly (seconds to a few minutes) to enable frequent execution.
- Bug Detection Rate: Good unit tests identify issues early, reflected by fewer bugs found in later testing stages or production.
- Maintainability: Tests should remain easy to update as code evolves, facilitating ongoing maintenance.
Conclusion
Effective unit testing is more than just a technical task—it’s a vital discipline that strengthens your development process. By adopting best practices, incorporating advanced strategies, leveraging suitable tools, and building a strong testing culture, developers can deliver reliable, maintainable, and high-quality software confidently and consistently.
Mastering unit testing ultimately makes you a better developer, your codebase healthier, and your end-users happier.