What is Node.js?
Node.js is an open-source, cross-platform runtime environment designed to allow developers to execute JavaScript code outside the confines of a web browser. Introduced by Ryan Dahl in 2009, Node.js was built on Google Chrome’s V8 JavaScript engine and revolutionized the way developers approached server-side programming. Traditionally, JavaScript was a language confined to client-side scripting in browsers, but Node.js extended its capabilities to server environments. This innovation created a unified programming environment for building scalable, fast, and modern web applications.
Key Features of Node.js
1. Asynchronous and Event-Driven
Node.js is built around an event-driven, non-blocking I/O model, enabling multiple operations to run simultaneously. Instead of waiting for tasks like database queries or file reads to complete, Node.js efficiently processes other tasks, making it ideal for high-traffic, real-time applications.
2. Single-Threaded Architecture
Node.js uses a single-threaded event loop to handle multiple concurrent client requests efficiently. By delegating complex tasks to background threads, it avoids issues like thread-locking, while maintaining lightweight and responsive server performance.
3. Cross-Platform Compatibility
Node.js works seamlessly across Windows, macOS, and Linux. Developers can write code once and deploy it on multiple platforms, simplifying development for distributed teams or diverse deployment environments.
4. Extensive NPM Ecosystem
The Node Package Manager (NPM) is a vast repository of over one million open-source packages. It provides pre-built modules for tasks ranging from authentication to logging, enabling faster development with reusable components.
5. High Performance and Scalability
Powered by the V8 engine, Node.js compiles JavaScript into optimized machine code, delivering fast execution. Its clustering feature supports horizontal scaling, allowing applications to handle increasing user demands.
Advanced Features of Node.js
1. Streams and Buffers
Streams process data in chunks, enabling efficient handling of large files like videos without consuming excessive memory. This is ideal for applications such as video streaming and file uploads. Buffers complement streams by managing raw binary data, ensuring smooth data manipulation in Node.js applications.
2. WebSockets
WebSockets enable persistent, bi-directional communication between client and server, unlike traditional HTTP. This is perfect for real-time applications like chat systems, live updates, and multiplayer games, allowing instant communication without frequent polling.
3. Clustering
Clustering allows Node.js applications to utilize multiple CPU cores by running parallel instances. This improves performance and scalability, making it ideal for handling high-traffic workloads by distributing requests across available resources.
4. Built-In Debugging Tools
Node.js includes tools like node inspect
for debugging directly from the terminal and supports third-party tools like Visual Studio Code. These tools simplify tracing and fixing issues, ensuring cleaner and more efficient code.
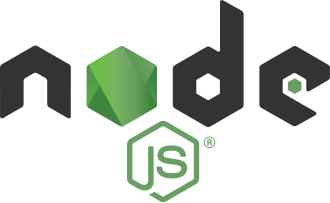
Why Node.js? Detailed Advantages
1. Fast Execution
The V8 engine used by Node.js translates JavaScript directly into native machine code, bypassing traditional interpretation overhead. As a result, Node.js applications are known for their high speed. Coupled with its asynchronous processing, applications built with Node.js can efficiently manage multiple tasks, reducing latency and improving user experiences. This speed is especially crucial for applications requiring rapid responses, such as financial systems, gaming platforms, or online stores.
2. Unified Development Environment
Before Node.js, developers often needed to use separate languages for frontend and backend development (e.g., JavaScript for the frontend and PHP, Python, or Ruby for the backend). Node.js allows developers to use JavaScript universally, promoting consistency in coding styles, reducing context-switching between languages, and making collaboration between frontend and backend teams smoother. This unification accelerates development cycles and simplifies the learning curve for new developers.
3. Active Community Support
Node.js is supported by a vibrant global community of developers, enthusiasts, and organizations. This community ensures a steady stream of updates, bug fixes, and security patches. Forums like Stack Overflow, GitHub repositories, and dedicated Node.js conferences provide a wealth of resources, enabling developers to troubleshoot problems and adopt best practices easily.
4. Real-Time Applications
Node.js excels in applications requiring real-time communication, such as chat applications, online gaming, and collaborative tools. The event-driven architecture ensures that changes made by one user can be instantly reflected across all connected clients without delays. For example, collaborative platforms like Google Docs can implement real-time synchronization using WebSockets or similar protocols in Node.js.
5. Rich Ecosystem
The extensive ecosystem of libraries and tools in NPM makes Node.js highly adaptable. Developers can quickly integrate third-party libraries for tasks like authentication, image processing, or email notifications. This ecosystem allows developers to focus on application logic rather than reinventing common functionalities, saving time and effort.
6. Microservices Architecture
Node.js is particularly well-suited for building microservices. Its lightweight nature and ability to handle multiple asynchronous operations make it ideal for developing and deploying small, independent services that communicate over lightweight protocols like HTTP. This approach enhances scalability, maintainability, and fault tolerance, as individual services can be updated or scaled without affecting the entire system.
How Node.js Works: An In-Depth Look
Node.js’s architecture is centered around three core components:
1. V8 JavaScript Engine
Google’s V8 engine, developed for Chrome, powers Node.js. It compiles JavaScript into machine code for direct execution by the CPU, bypassing the slower interpretation phase typical in older engines. This results in fast execution and performance optimization, particularly for applications with high computational demands.
2. Libuv
Libuv is a C library that provides Node.js with its non-blocking I/O capabilities. It serves as the backbone for handling operations such as file system interactions, networking, and DNS lookups. Libuv works in conjunction with the event loop, distributing tasks to a thread pool when necessary, ensuring that the main thread remains responsive.
3. Event Loop
The event loop is the beating heart of Node.js. It operates on a single thread, continuously monitoring a queue of events and delegating tasks to the appropriate handlers. When an I/O operation is completed, the event loop executes the associated callback. This mechanism ensures that Node.js can handle thousands of concurrent requests with minimal resource usage.
Common Use Cases for Node.js
1. Real-Time Applications
Node.js’s event-driven and non-blocking architecture makes it perfect for real-time applications. For instance:
- Chat Applications: Platforms like Slack or WhatsApp require instantaneous message delivery across users, which Node.js achieves using WebSockets.
- Online Gaming: Multiplayer games rely on real-time updates to ensure that players see synchronized game states.
2. API Development
Node.js is a go-to choice for building RESTful and GraphQL APIs. Its lightweight design and asynchronous capabilities ensure efficient handling of multiple client requests. Popular frameworks like Express.js simplify API creation, while libraries like Axios and Fetch enhance client-server communication.
3. Streaming Services
Applications like Netflix and Spotify benefit from Node.js’s ability to handle data streams efficiently. Instead of downloading large files, Node.js streams data in chunks, reducing memory usage and improving performance.
4. Internet of Things (IoT)
Node.js is increasingly used in IoT applications due to its lightweight and event-driven design. It can manage real-time data from devices like sensors, cameras, and wearables, enabling responsive and scalable IoT ecosystems.
Best Practices for Using Node.js
1. Use Asynchronous Code
Node.js operates on a single-threaded event loop, making it crucial to avoid blocking operations. Using asynchronous functions ensures that other tasks can continue without delays. Promises and async/await
make asynchronous code more readable and easier to debug compared to traditional callback-based approaches. For example, instead of nesting callbacks for multiple asynchronous operations, async/await
lets you write clean, sequential code that behaves predictably, improving maintainability.
2. Optimize for Performance
Performance optimization is key to building scalable Node.js applications. Tools like PM2 not only manage application processes but also support features like clustering, which leverages multiple CPU cores to handle high workloads efficiently. Additionally, profiling tools such as Node.js Performance Hooks can help identify bottlenecks in your application, allowing you to make data-driven optimizations for better speed and resource utilization.
3. Secure Your Application
Security is critical in any application, and Node.js offers various tools to help safeguard your app. Using packages like Helmet helps secure HTTP headers, mitigating risks such as clickjacking and XSS attacks. JSON Web Tokens (JWT) provide a robust way to implement secure authentication mechanisms, while tools like Snyk or npm audit help identify and address vulnerabilities in dependencies. Regularly updating libraries ensures your application stays protected against known security flaws.
4. Leverage NPM Modules
NPM provides access to a vast ecosystem of packages, significantly accelerating development by offering pre-built solutions. However, it’s essential to vet third-party modules before use. Check the module’s popularity, maintenance status, and security vulnerabilities to avoid introducing unnecessary risks. Additionally, keeping track of dependency versions and licenses helps maintain a secure and compliant codebase while benefiting from the vast resources NPM offers.
Tools and Frameworks for Node.js
Express.js is a minimal and flexible framework for building web applications and APIs. It simplifies tasks like routing, middleware integration, and handling HTTP requests and responses. With its unopinionated design, developers have the freedom to structure applications as they see fit. Express is widely used for creating RESTful APIs and single-page applications, and its extensive ecosystem of plugins and middleware makes it suitable for projects of any size.
NestJS is a progressive framework designed for building scalable, enterprise-grade backend applications. Built on top of Express (or optionally Fastify), it adopts a modular architecture inspired by Angular, promoting code reusability and maintainability. NestJS supports TypeScript out of the box, offers powerful dependency injection, and integrates seamlessly with libraries for databases, authentication, and more. It’s an excellent choice for teams looking to implement complex, large-scale solutions with clean and testable code.
Socket.IO is a powerful library that enables real-time, bi-directional communication between clients and servers. Unlike traditional HTTP, which requires constant polling for updates, Socket.IO establishes a persistent connection, allowing instant message exchange. It’s ideal for applications like live chat, gaming, and real-time analytics dashboards. Additionally, Socket.IO handles reconnections and cross-platform compatibility, making it easy to implement responsive and reliable real-time features.
PM2 is a process manager designed to enhance the performance and reliability of Node.js applications. It automates common tasks such as starting, stopping, and restarting applications, and includes features like log management, performance monitoring, and clustering. Clustering allows PM2 to utilize multiple CPU cores, improving application scalability. PM2’s monitoring dashboard provides insights into application metrics, helping developers identify and resolve performance issues quickly. It’s a vital tool for maintaining uptime in production environments.
Conclusion
Node.js has reshaped the landscape of server-side programming by extending JavaScript’s capabilities beyond the browser. Its non-blocking, event-driven architecture, combined with its speed and scalability, makes it a powerhouse for building modern applications. From real-time systems and APIs to microservices and IoT solutions, Node.js offers unmatched versatility.
With a vibrant ecosystem, robust community support, and compatibility with modern development practices, Node.js continues to be a leading choice for developers worldwide. Whether you’re a beginner exploring backend technologies or a seasoned developer scaling enterprise systems, Node.js provides the tools, flexibility, and performance needed to succeed in today’s fast-paced digital world.